If you have a list of objects and want to store them in an array, you can easily do it by using JavaScript.
To Push an Object inside an Array there are many options and methods available in JavaScript like splice and unshift methods, spread operator, also, etc.
The most common and easiest way to do this is by using the JavaScript push() method, here is how,
Create an empty array
and create your object
, after that push your object into that empty array
using the push() method
. for example,
Here is an example:
// Create an array
let myArray = [];
// Create objects
let obj1 = { name: 'Alice', age: 25 };
let obj2 = { name: 'Bob', age: 28 };
// Push objects into the array
myArray.push(obj1);
myArray.push(obj2);
// Display the array
console.log(myArray);
Output:
[ { name: 'Alice', age: 25 }, { name: 'Bob', age: 28 } ]
In today’s article, we will see how to push an object to an array method with the push() method in an easy step-by-step.
Benefits of pushing objects into an array
Pushing objects into an array is very common and has some amazing advantages like
Organization and structure, built-in methods like push, pop, map, filter and reduce, flexibility, adaptability, efficiency, and many more.
Follow all the steps given below carefully.
How to JavaScript Push Object to Array using steps
Step 1: Create an empty array
The first step is to create a row or empty array
,
inside your JavaScript file, Create a variable by giving a name and also add an empty square bracket []
Example:
let myArray = [];
The above example code has a let
variable named myArray
and empty brackets []
Step 2: Make an object
Once you have created your empty array then in this step we will create our object
If you have already created your object, you can skip this step and go to step 3.
Simply you can create your object methods by adding your data to your object Here is my object with an example:
let myObject = {
name: "John",
age: 30,
};
As you can see in the above example, the object is named `myobject
` which contains `name
` and `age
`
Step 3: Push the object to the array
After creating the object let’s push the object into an array,
As I said we are going to use the JavaScript push() method for an object so the object will be inside the array method
Example:
myArray.push(myObject);
In the above example, I have my empty array and I have invoked a push() method after which I have added my object inside the brackets `()`
The push method now automatically pushes my object inside the array.
Step 4: Output in the console
After following all the steps, now try console.log
on your browser.
To preview it in the console first write the code, call console.log()
in your own space, and see the output. Here’s an example,
Example:
myArray.push(myObject);
After running this code there will be only one object in `myArray
`, you can also add more objects
to the array
in the same way
Here is the Output:
The final output is now inside the object array
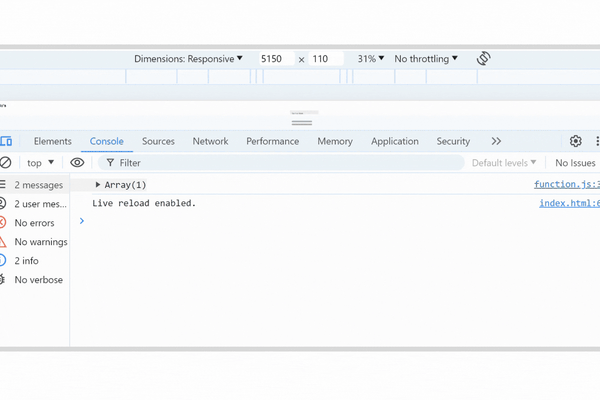
As you can see now, I have my object inside the Array method, you can also print it in the document using the`document.write
`
I hope this article helps you push your object into an array method.
Related Posts: